Registration
Learn how to set up the registration form, submit handler, and the OwnId integration
Registration works with your existing user registration form to add new users or enroll new devices for existing users.
Prerequisites
To get the most out of this guide, make sure to do the following tasks first:
- Complete the backend integrations (see Build Server-Side Endpoints).
- Complete the Login integration (see Login).
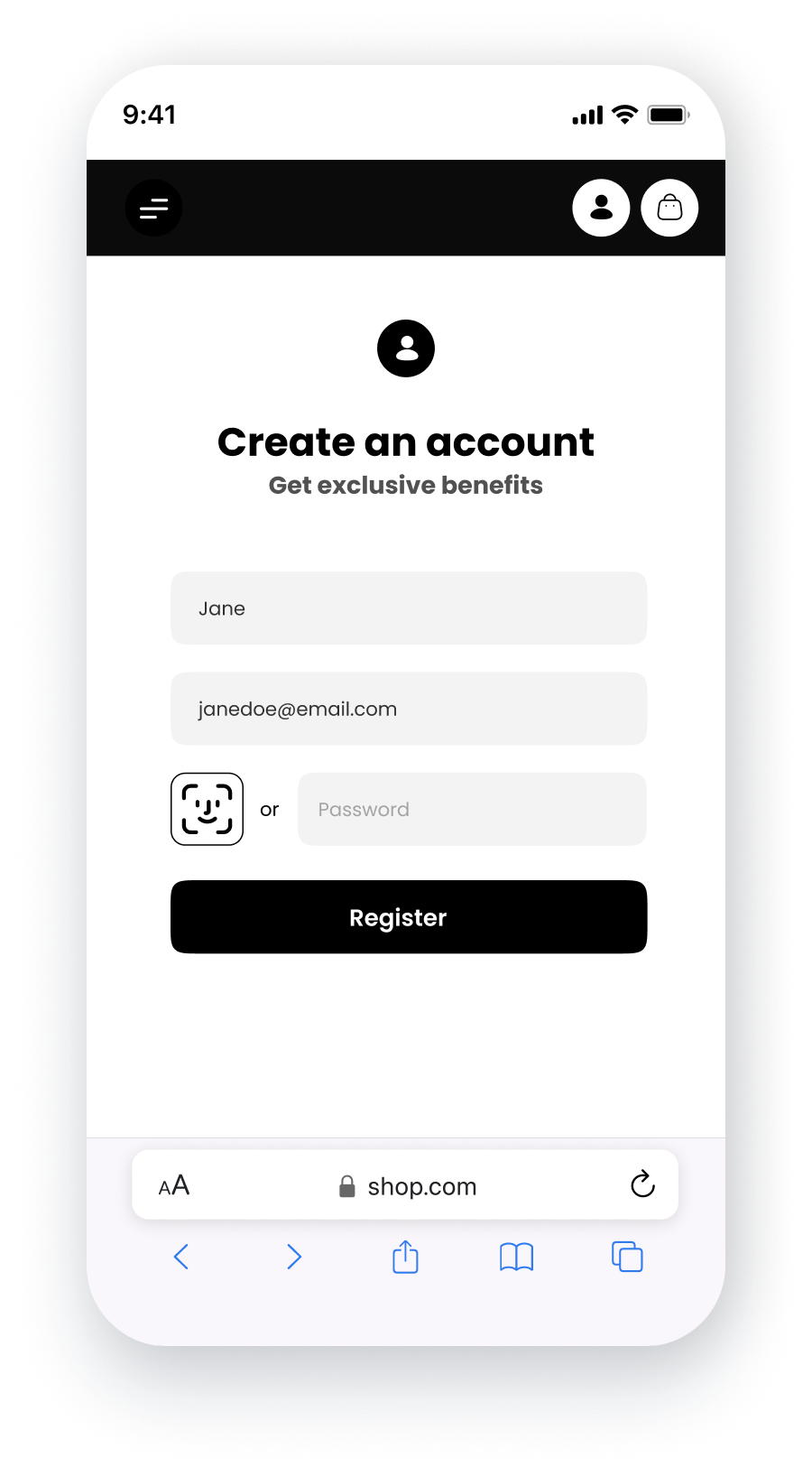
The instructions on this page assume you have already installed and referenced the OwnID SDK at the top of your page as described in Steps 1 and 2 of Login.
Integrating Registration
Implement the event handler, and OwnID registration integration with references to the password and user id fields in your existing form.
Add the Widget to your Registration Form
The SDK register method is used to integrate the registration journey. It references the fields in your existing registration form. It also renders the OwnId widget by automatically referencing your field Ids to calculate its position in the DOM.
The sample registration forms in the snippets below are vanilla examples, shown here only to confirm the implementation pattern of form fields in SDK methods.
Implement register Method
In the ownid method:
-
Enter “register” for the method name.
-
Assign the form field (as a DOM element) you use as the password to the
passwordField
parameter. -
Assign the form field (as a DOM element) you use as the login id to the
loginIdField
parameter. -
Set the
passwordFieldBinding
parameter totrue
if you want to force the OwnID platform to automatically create a random strong password. The value isfalse
by default. -
Assign an error function as desired in the
onError
parameter. -
Configure the
onRegister
event to copy theownIdData
object to a local variable. When submitting the registration form, you must send that object to your backend and store it in theownIdData
field you created during Preparation.
Use the code snippets below, and check the embedded comments, to model your implementation of the submit handler and the SDK register method.
That’s it!
Your users can now register for a new account and leverage other user journeys you implement.
Before going live make sure you check out the following the next steps.
Next Steps
Ready to deploy?
Was this page helpful?